Object Modification
Objects are the fundamental building blocks of any object-oriented programming language which includes Visual Basic .Net (VB.Net), the main programming language used in UiPath. Everything that is done in UiPath involves modifying or manipulating objects.
What are Objects?
An object represents a single piece of data. For example, a door, table, and a car are all objects in the real world. In programming, an object can be a variable, a data type, or even a data collection. By using properties, methods, and functions as discussed below, you can modify an object or even a collection of objects.
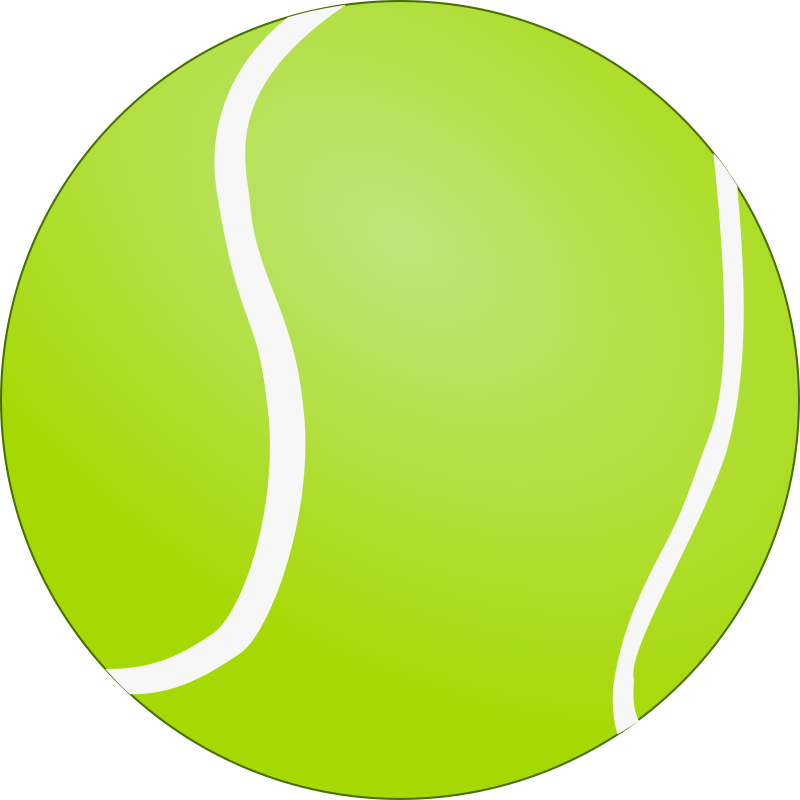

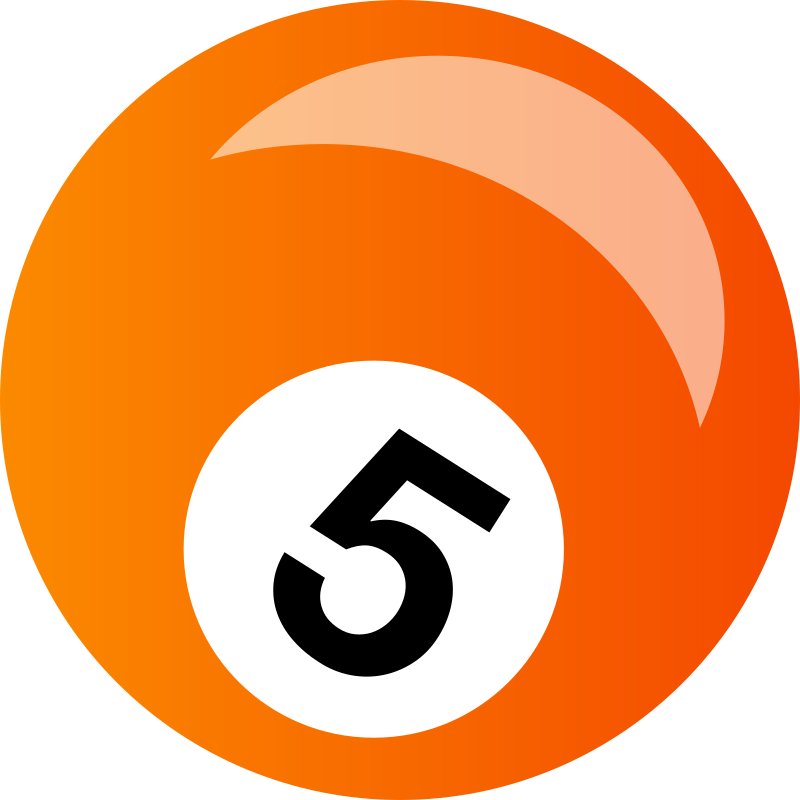
What is a Property?
A property is a characteristic or attribute of an object. For example, the properties of a car are the color, model, make, and year it was made.
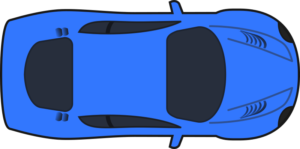
Car.Color = Blue
Properties allow us as developers to obtain further information about objects that are used within an automation.
For example, if you developed a robot to read an email and reply to the sender, you can use the properties of the email object to obtain the senders email address.
Properties can be used inside any UiPath Activity, just like a variable would be. Properties are written out after the variable as a period (.) followed by the name of the property being used. Properties can be shown by the example below:

In the above Assign activity, we are using the Length property of the String data type to determine how many characters are in the string variable called stringVar. The output of this assign is an Integer variable called intVariable.
Some properties of objects allow you to add parameters to help narrow down your results. Any parameters added to a property are written within a parenthesis following the property name. Properties with parameters can be shown by the example below:

In this Assign activity, we are using the Chars property of the String data type to determine the character sitting at a specified index in the string variable called stringVar. Using the parameter of 2 in parenthesis after the property allows us to specify at which index we want the robot to look at. The output of this assign is an Integer variable called charVar.
What is a Method?
A method is an action that an object can perform. For example, the methods of a car would include driving, parking and braking.

Car.Drive = Faster
Methods allow us as developers to modify objects or variables that are used in our automations. For example, if you developed a robot to read the time and write it into a log, you would need to use a Method to convert the DateTime value into a String data type.
Methods can be used inside any UiPath activity, just like a variable would be. Methods are written out after the variable as a period (.) followed by the name of the method being used. A Method can be shown by the example below:
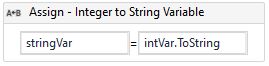
In this Assign activity, we are using the ToString method of the String data type to convert the integer variable, intVar to a string variable named stringVar.
Just like properties, some methods allow you to add parameters to help narrow down your results. Any parameters added to a method are written within a parenthesis following the method name. Methods with parameters can be shown by the example below:

In this Assign activity, we are using the Contains method of the String data type to determine if the string variable contains another string inside of it. In this instance, we are looking to see if stringVar contains the string “Hi”. If successful, the boolean variable, named boolVar will become True, otherwise it will become False.
What is a Function?
A Function is a self-contained block of code that is used to perform a single action. Functions have a whole plethora of uses, but here, we will only be discussing its capability to modify objects. Functions, just like methods, are a set of instructions that perform a task. The main difference between the two is that a function does not need to be associated with an object, whereas a method does.
Functions can be used within UiPath to accomplish tasks such as modifying variables, converting data types, and even containing small blocks of code.
Functions can also be used inside any UiPath activity, just like a variable would be. Functions are written by the function name followed by a set of two parentheses with the variable name within them. Functions can be shown by the example below:

In this Assign activity, we are using the Cbool() function to convert the string variable named stringVar into a Boolean variable called boolVar.
Conclusion
To summarize, you can think of Properties as qualities of an object and Methods as actions an object can perform. Functions are also useful to modify objects, but have more advanced or specific uses.
Not all methods or properties work for all data types. There are specific methods and properties for each data type. For more information, you can check out each of the methods and properties assigned to the data types listed here.